Knowledge Base - How to create your own APP with Xamarin (iOS) and RadaeePDF PDF reader
- Install RadaeePDF-Xamarin demo from: https://github.com/gearit/RadaePDF-Xamarin
- Launch Xamarin Studio and click on New Project:
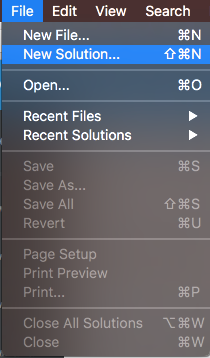
- Create an iOS App, select App then Single View App (iOS). Enter a project name (TestRadaee):
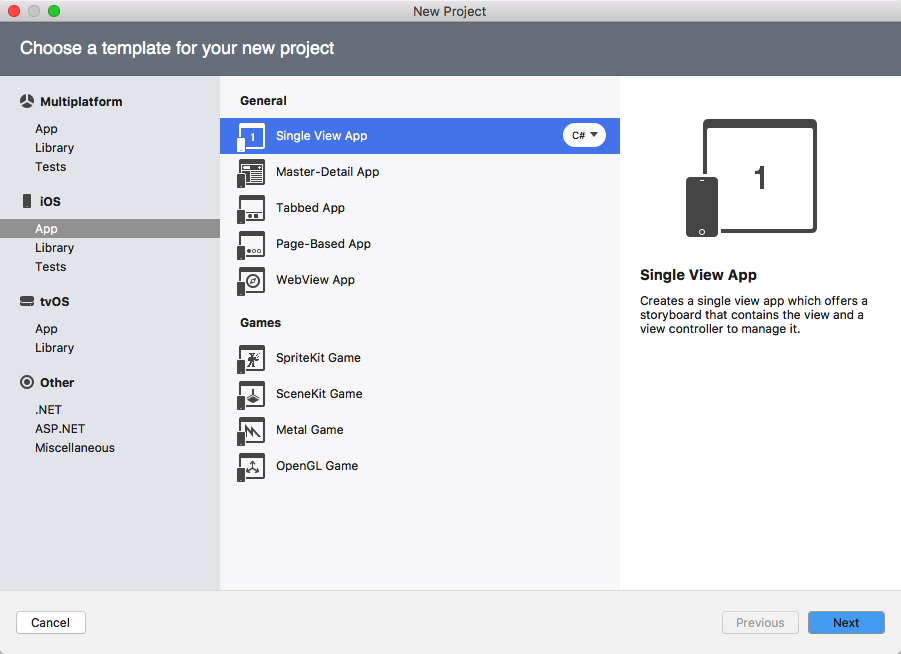
- Now add the Radaee base project as project dependency:
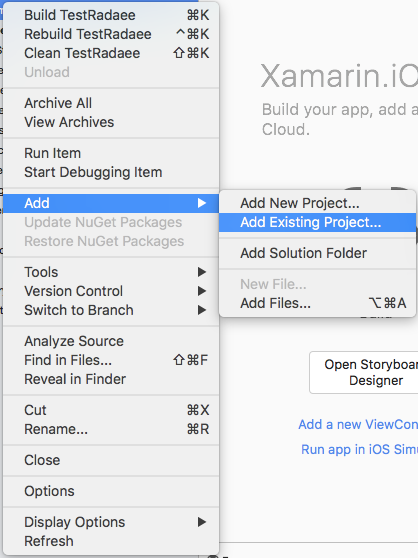
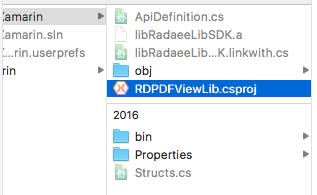
- Now add the project reference, right click on References->Edit References... :
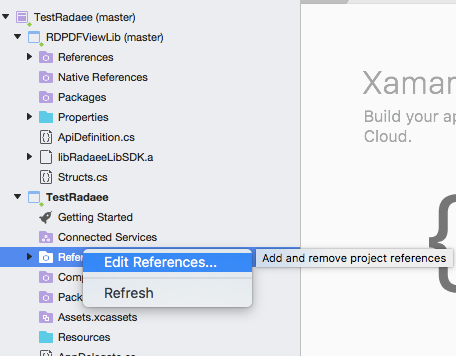
- And select RDPDFViewLib:
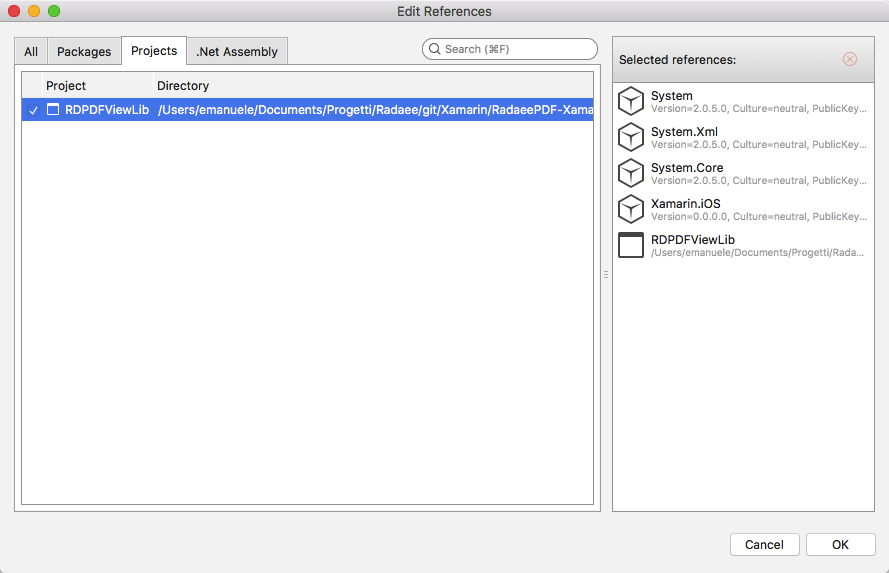
- Edit Info.plist and set the correct Bundle id (If you do not have an active license, please use "com.radaee.pdf.PDFViewer" as Bundle id, to be able to test all the SDK features)
- Add needed resources from demo project, right click on Resources->Add->Add Files from Folder...:
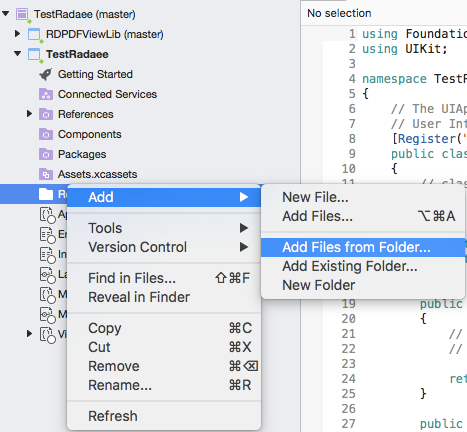
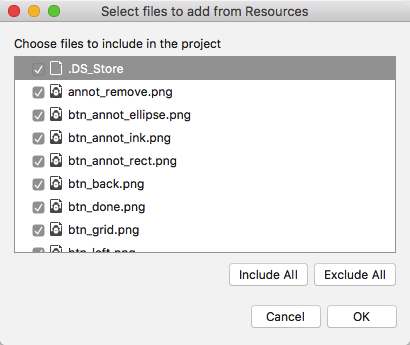
- Open TestRadaee->Main.storyboard, and add a navigation controller and abutton to open a pdf file:
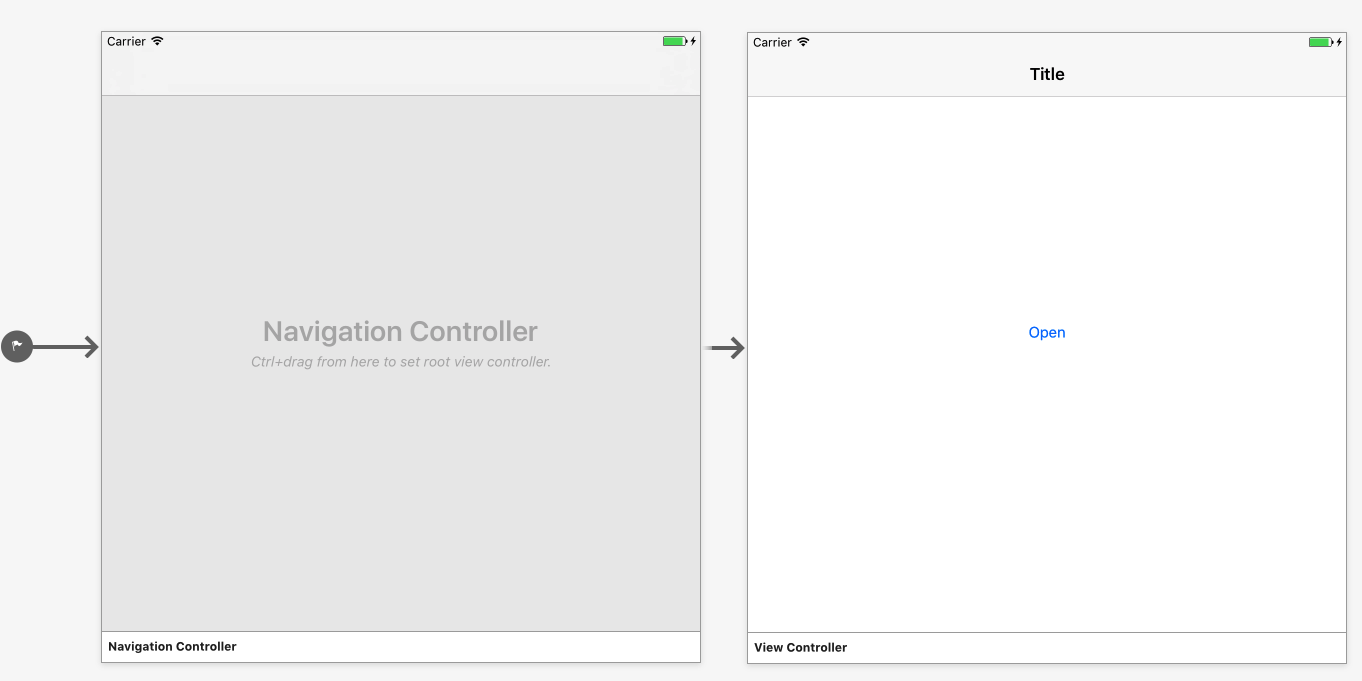
- Double click on button to create an action in ViewController.cs:
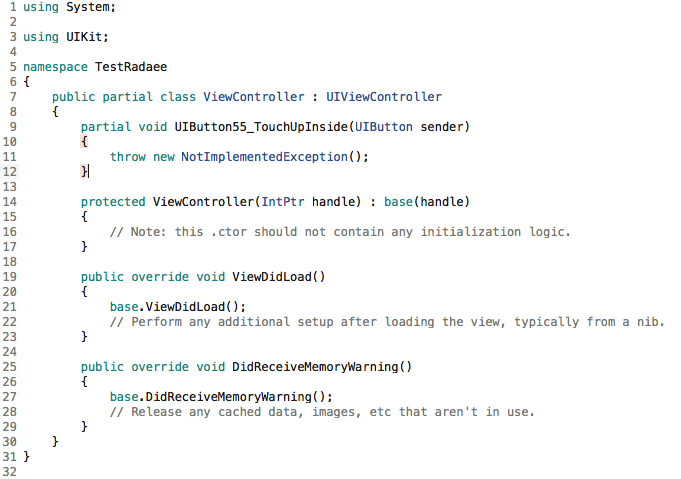
- Add RadaeeLib reference in ViewController.cs:
using RadaeeLib;
- Open TestRadaee->ViewController.cs and add RadaeeDelegate implementations to get callbacks from reader:
public class RadaeeDelegate : RadaeePDFPluginDelegate
{
RadaeePDFPlugin plugin;
public RadaeeDelegate(RadaeePDFPlugin plugin)
{
this.plugin = plugin;
}
public override void WillShowReader()
{
Console.WriteLine("will show reader");
}
public override void DidShowReader()
{
Console.WriteLine("did show reader");
}
public override void WillCloseReader()
{
Console.WriteLine("will close reader");
}
public override void DidCloseReader()
{
Console.WriteLine("did close reader");
}
public override void DidChangePage(int page)
{
Console.WriteLine("did change page {0}", page);
}
public override void DidSearchTerm(string term, bool found)
{
Console.WriteLine("did search {0}", term);
}
}
- Create the following ViewController properties:
RadaeeDelegate selector;
RadaeePDFPlugin plugin;
- Open TestRadaee->ViewController.cs and edit the button action with the following code to open a pdf file:
//Reader settings init
plugin = RadaeePDFPlugin.PluginInit;
//Activate license
plugin.ActivateLicenseWithBundleId("com.radaee.pdf.PDFViewer", "Radaee", "radaee_com@yahoo.cn", "89WG9I-HCL62K-H3CRUZ-WAJQ9H-FADG6Z-XEBCAO", 2);
//Set Callback for RadaeeDelegate
selector = new RadaeeDelegate(plugin);
plugin.SetDelegate(selector);
UIViewController controller = plugin.OpenFromAssets("test.pdf", "");
if (controller != null)
{
//show reader
this.NavigationController.PushViewController(controller, true);
}
else
{
UIAlertView alert = new UIAlertView();
alert.Title = "Error";
alert.Message = "PDF not found";
alert.AddButton("OK");
alert.Show();
}
- Now ViewController.cs should be something like:
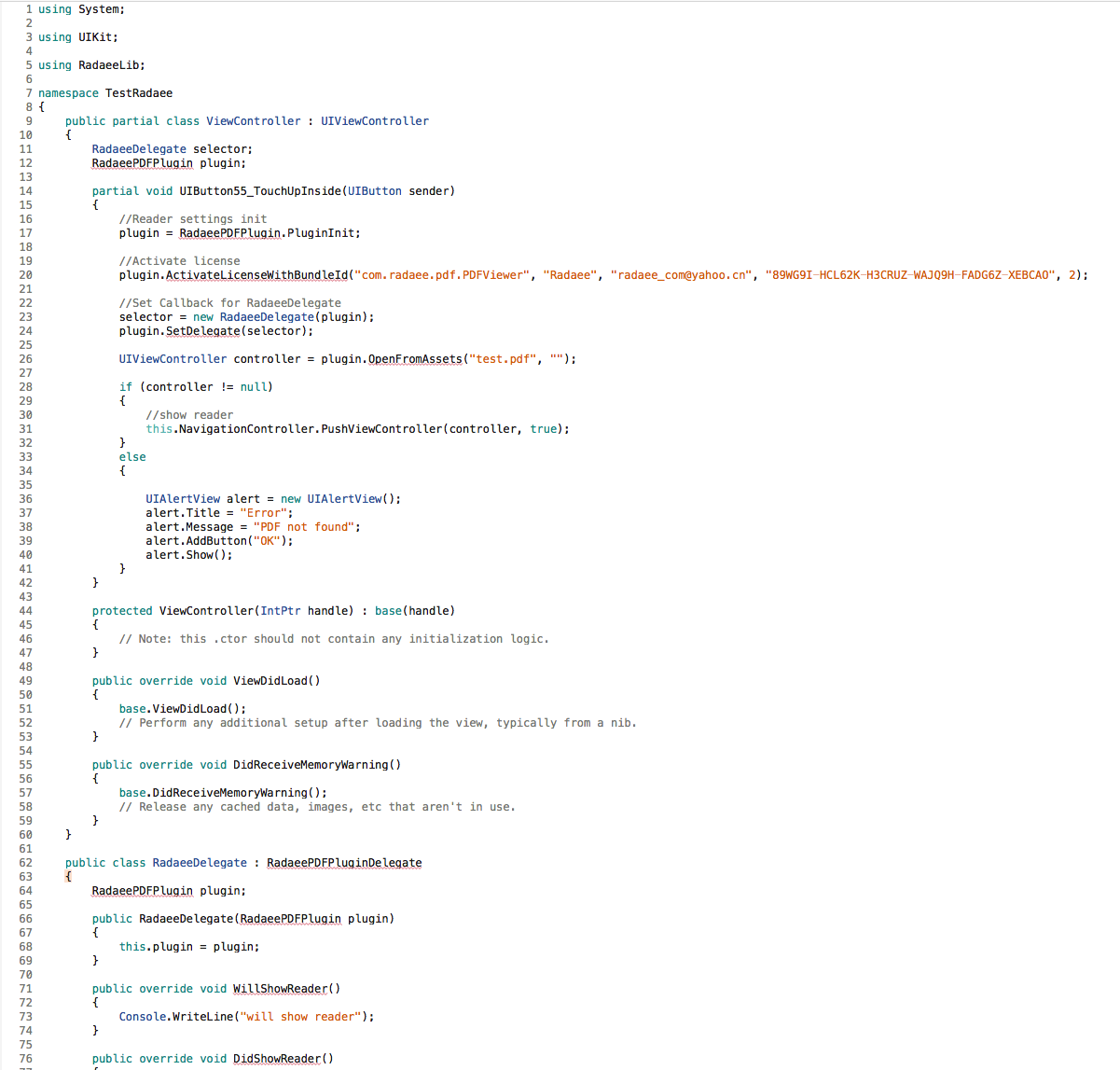
Applies To
RadaeePDF SDK for Xamarin
Details
Created : 2017-03-01 16:59:10, Last Modified : 2022-03-21 17:52:04